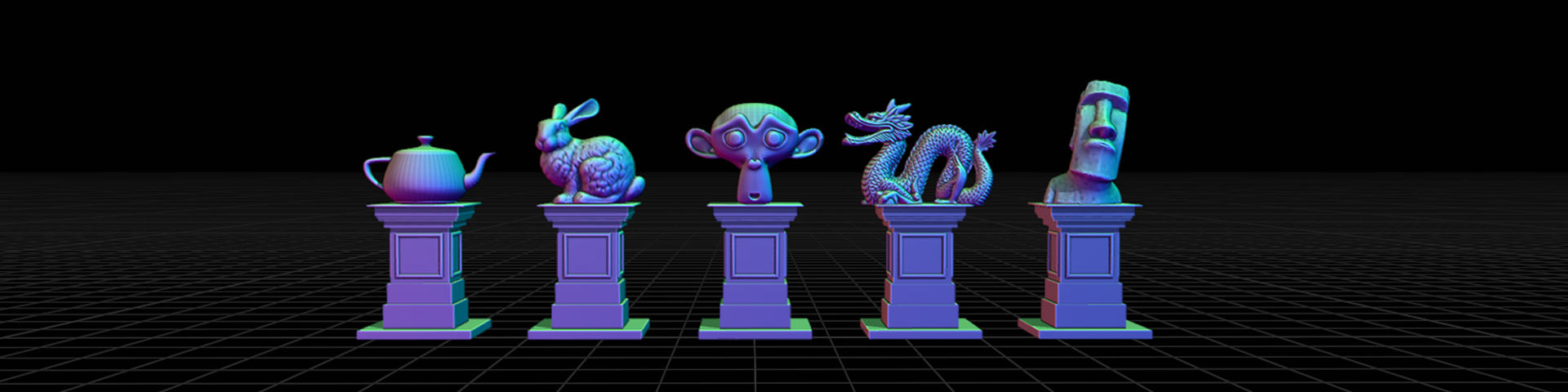
Throughout my 3D engineering classes at ESIEE Paris, I explored different aspects of graphics programming, including geometric computing, OpenGL rendering, ray marching techniques, and complex particle systems in Unity. You will find here my key findings and implementations in these areas.
Geometric Computing and Half-Edge Data Structures
During my "Geometric Modeling" classes I got to implement and experiment with the half-edge data structure, which provides an elegant way to traverse and manipulate meshes. I used this approach to load and parse .obj files in C++, compute face normals, extract silhouettes, and perform mesh triangulation. Later, I explored mesh simplification (decimation) techniques to reduce polygon count while preserving shape, and I implemented Catmull-Clark subdivision algorithm to generate smooth surfaces dynamically.
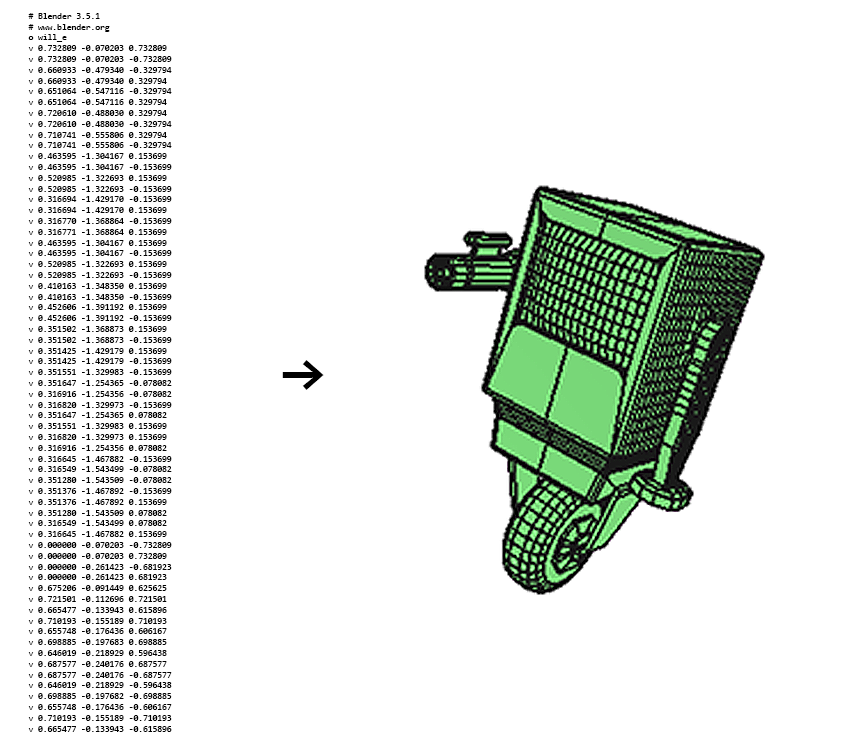
Loading an .obj file
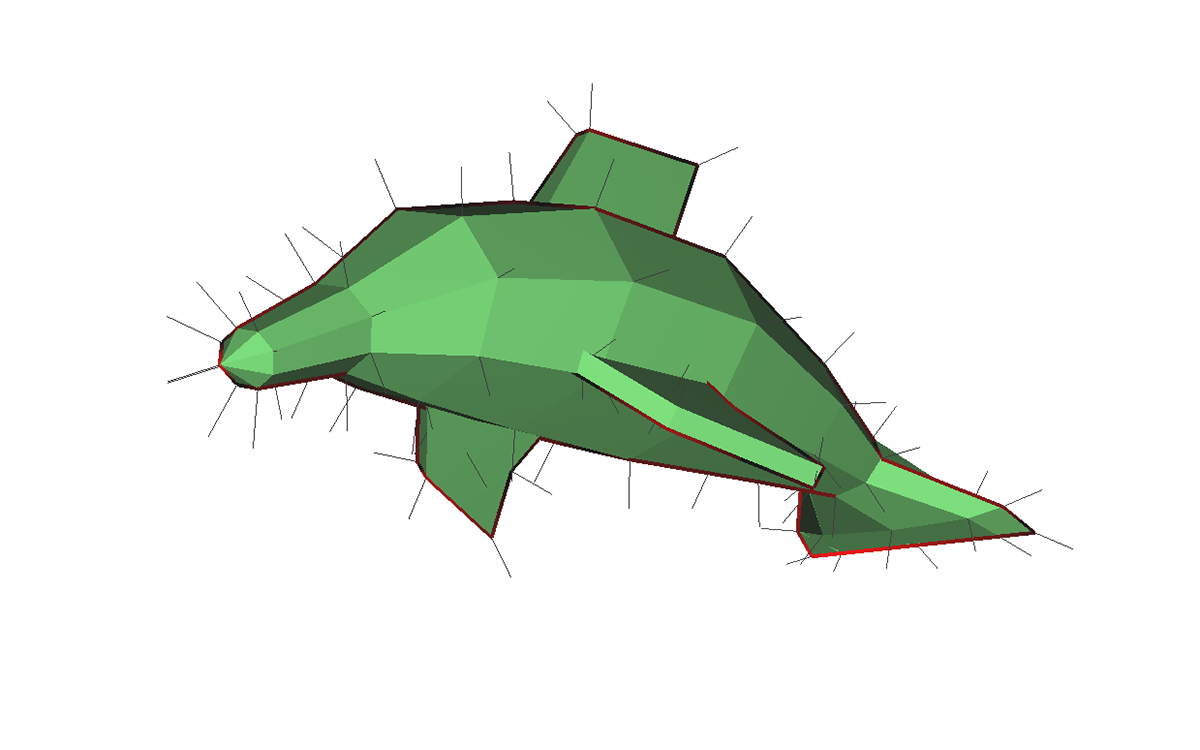
Normals viewing and mesh silouhette
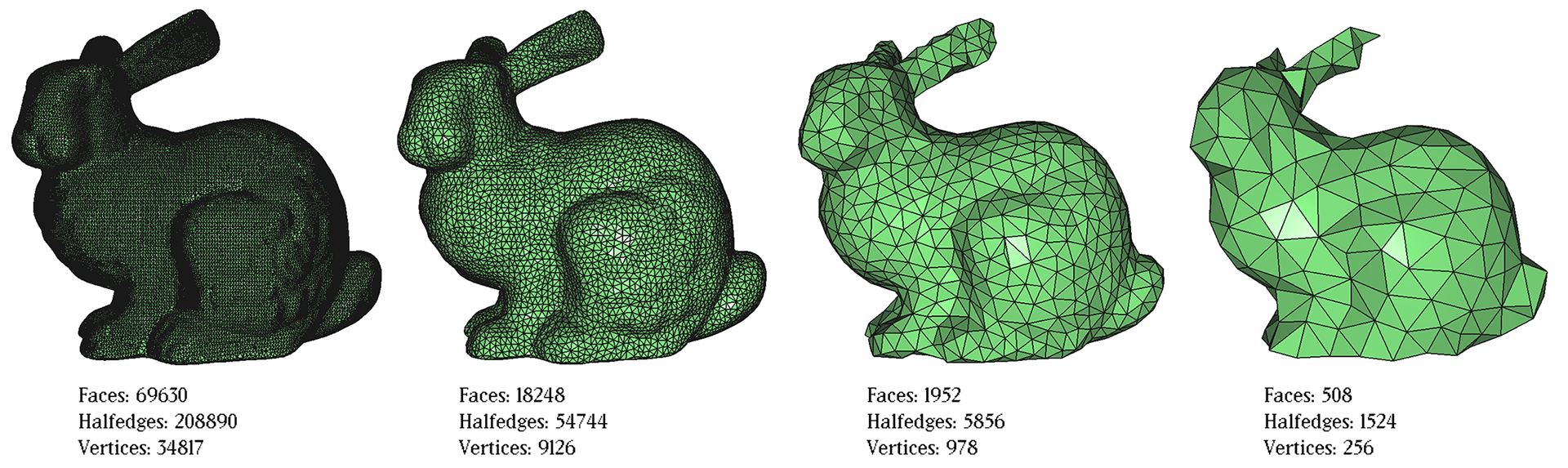
Mesh simplification (simple half-edge collapse decimation) on Stanford Bunny
Catmull-Clark Subdivision Surface Algorithm implementation using halfedge datastructure:
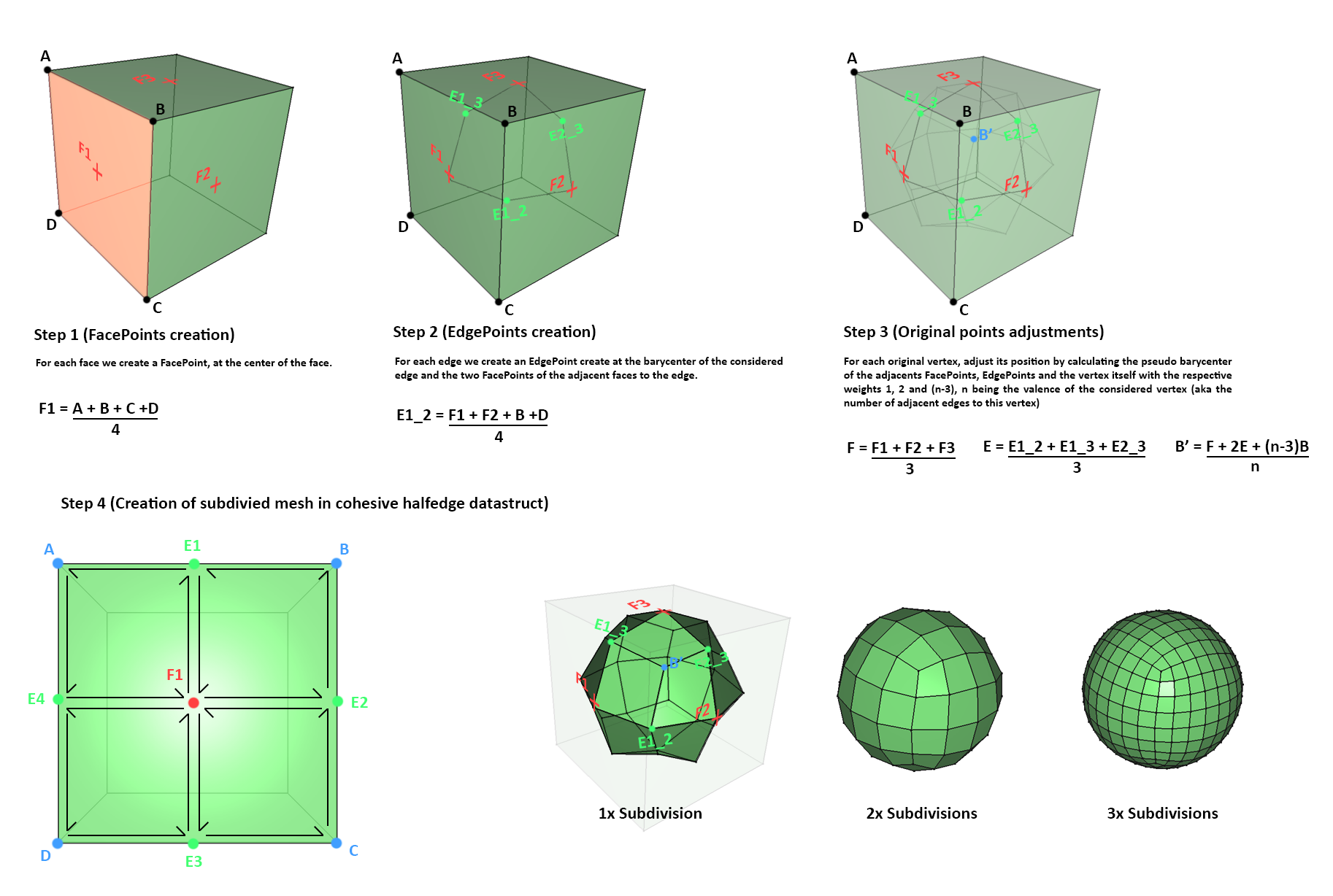
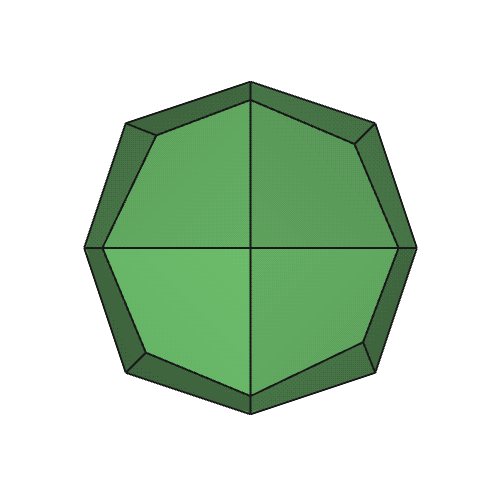
4x Catmull-Clark subdivisions on a cube
OpenGL Rendering Pipeline and Scene Viewer
During my second year I built an OpenGL viewer in C++ capable of loading multiple .obj models with their associated textures. The program reads material properties from .mtl files and applies Blinn-Phong shading to the scene.
I was able to experiment with the complete pipeline of OpenGL 2.0 and play along with all the different steps of it (vertex and fragment shader, rasterization, testing & blending etc)
All in all I ended up with a finished scene viewer, completed with a user interface (Dear ImGui), enabling real-time parameters changes.
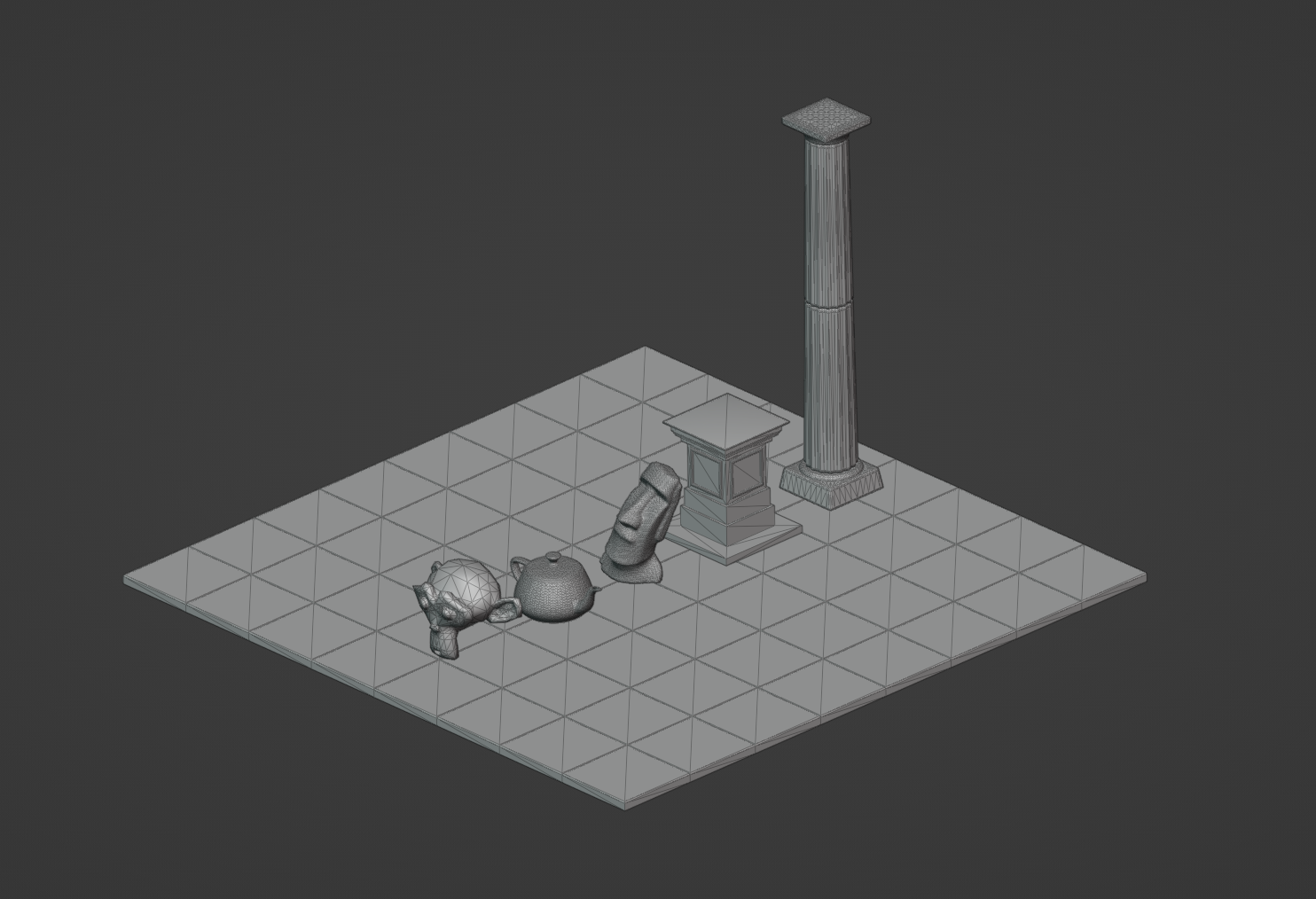
Scene objects to be imported in the OpenGL viewer
OpenGL Viewer demo
Experiments with Ray Marching
I started experimenting with ray marching during my second year at ESIEE Paris. My first project was a simple Lambert-shaded sphere, defined by its Signed Distance Function (SDF) and rendered in Unity using a compute shader. Building on this, I worked on volumetric cloud rendering, where clouds are generated using 3D noise functions and react dynamically to ambient lighting. This technique was developed for a Unity game, ensuring real-time performance and a convincing atmospheric effect.
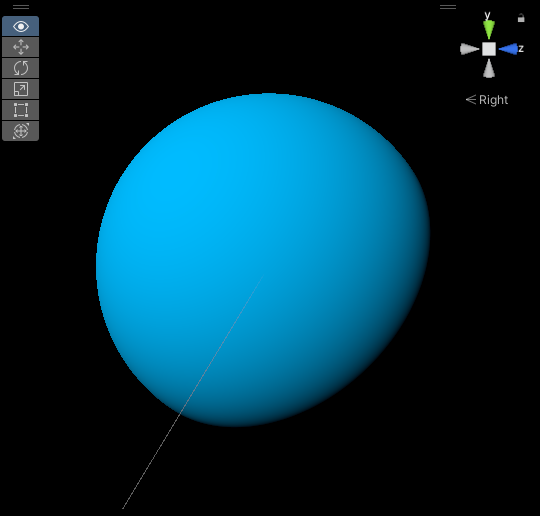
Ray Marching on a sphere SDF with Lambertian reflectance
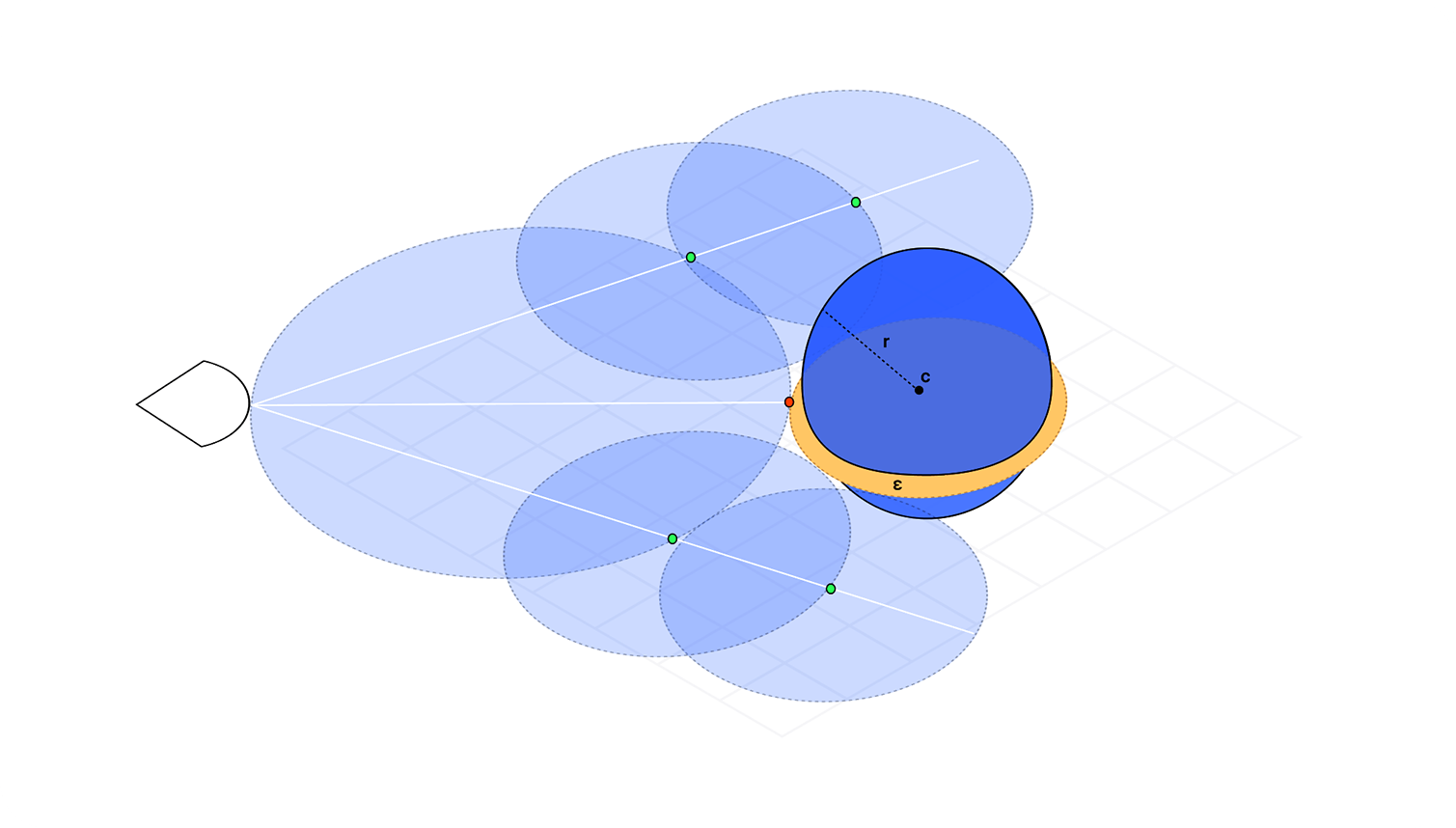
Ray Marching Diagram (©Maxime Heckel)
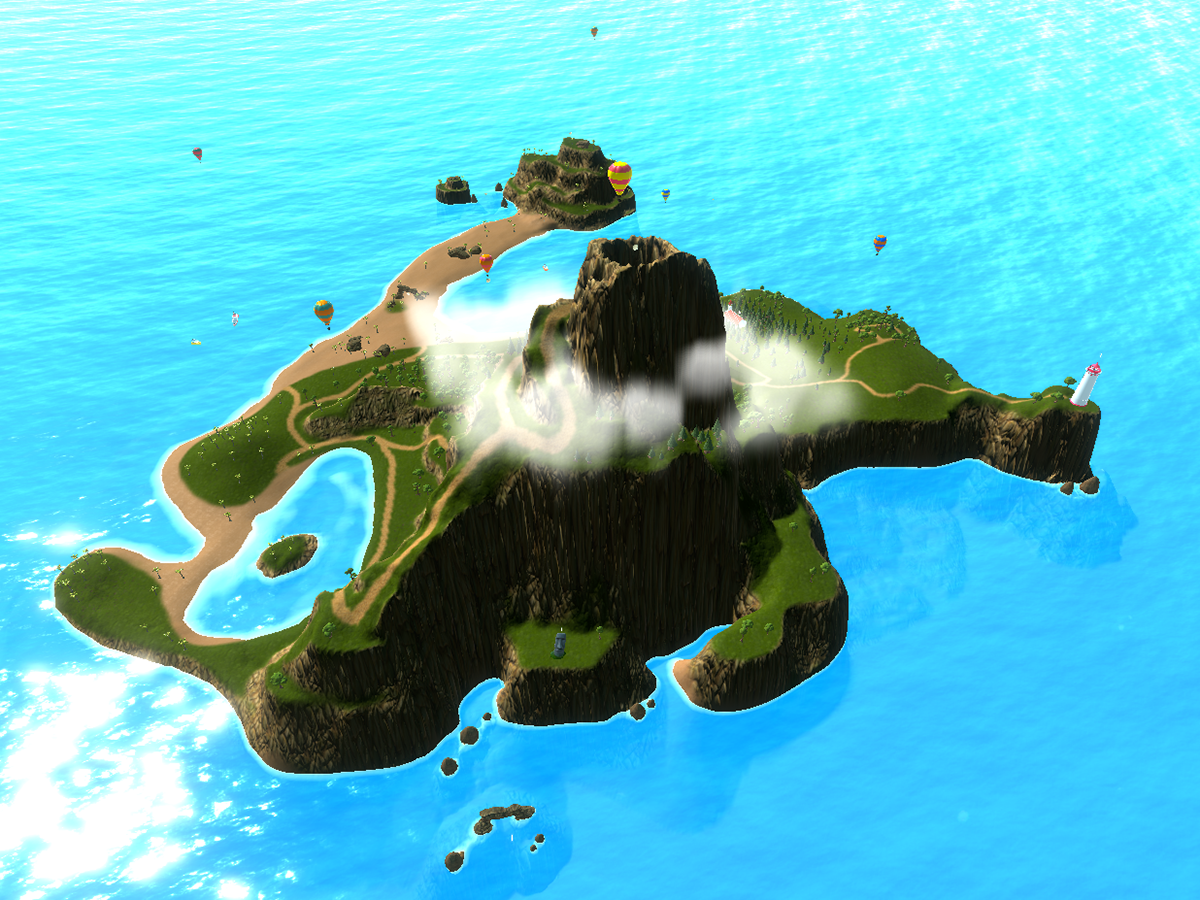
Top view of the game island with Ray Marching clouds around the peak
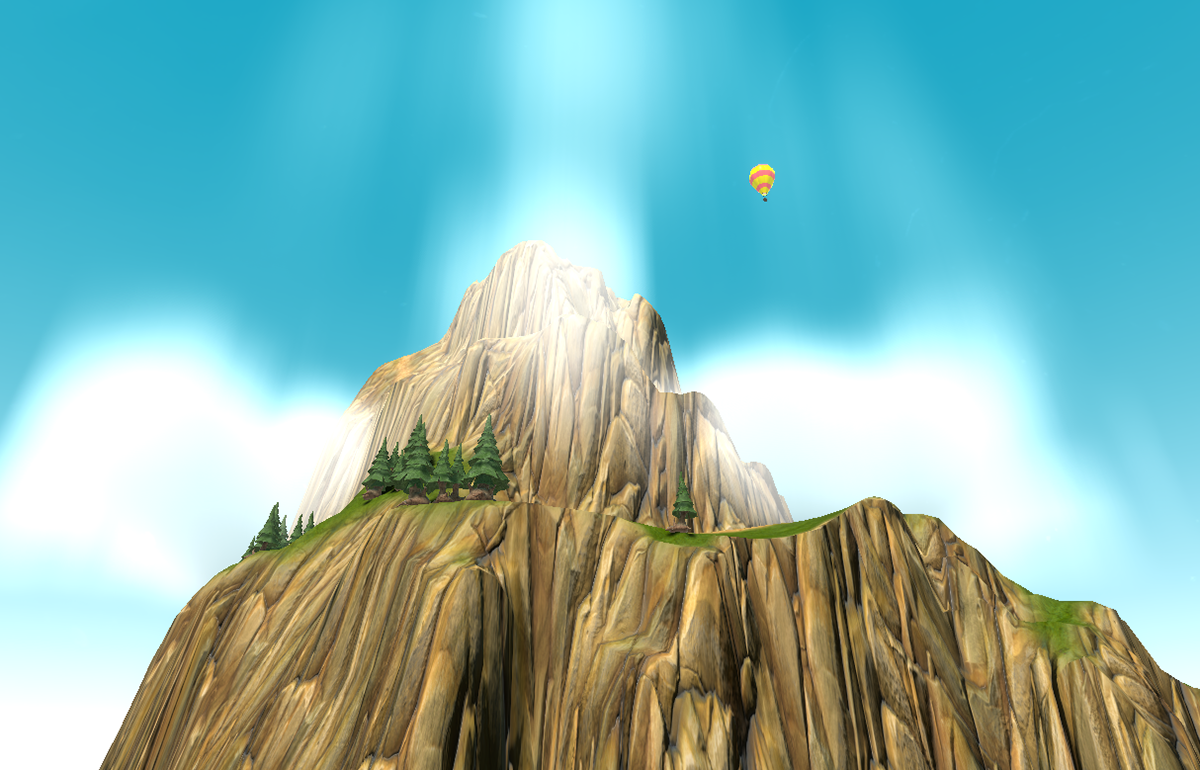
Clouds at day
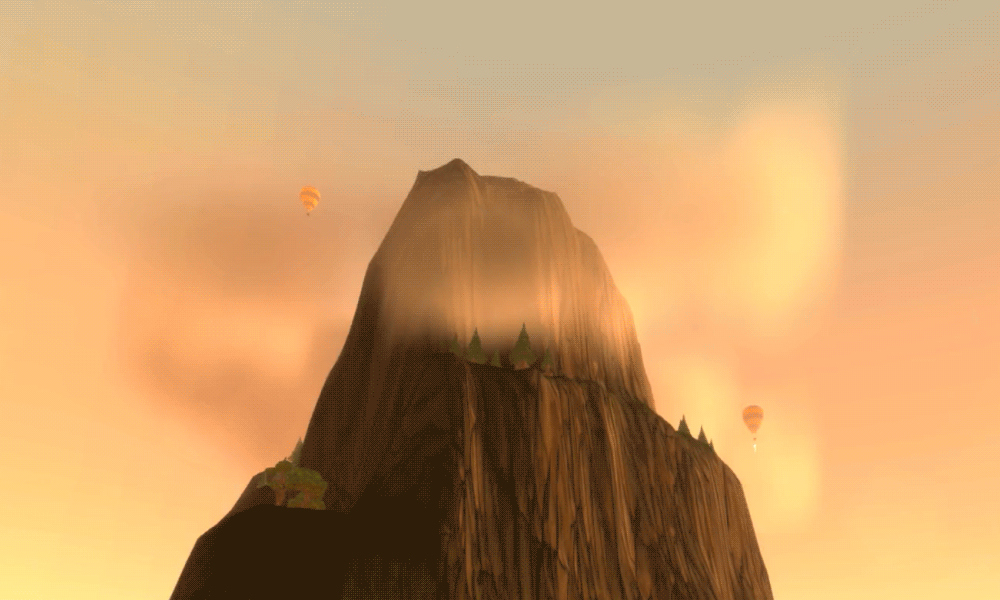
Clouds at dusk
Advanced Particle Systems in Unity
Exploring particles systems, I designed a fireworks simulation in Unity using GPU Events, enabling efficient large-scale particle interactions. Another experiment involved creating a water-landing animation for an airplane game, where the particles simulated stylized splash and turbulence effects upon contact with the water.
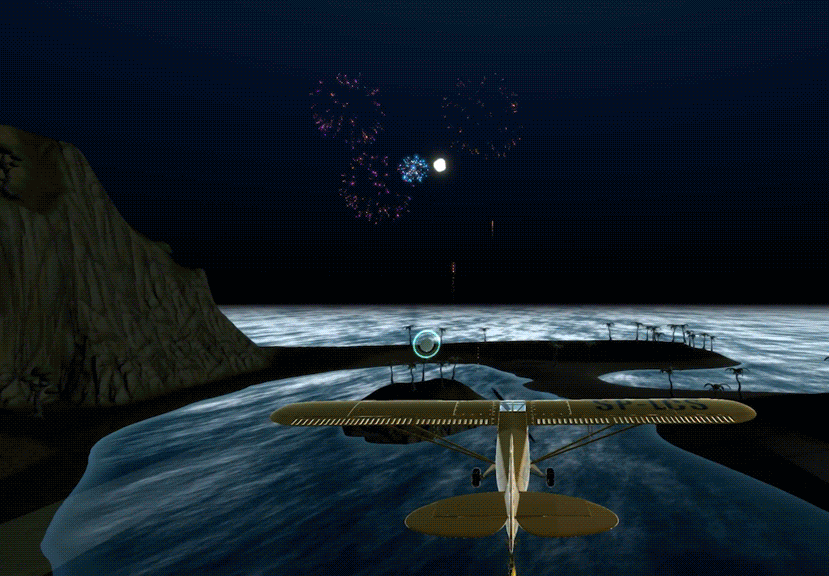
Fireworks in--game simulation
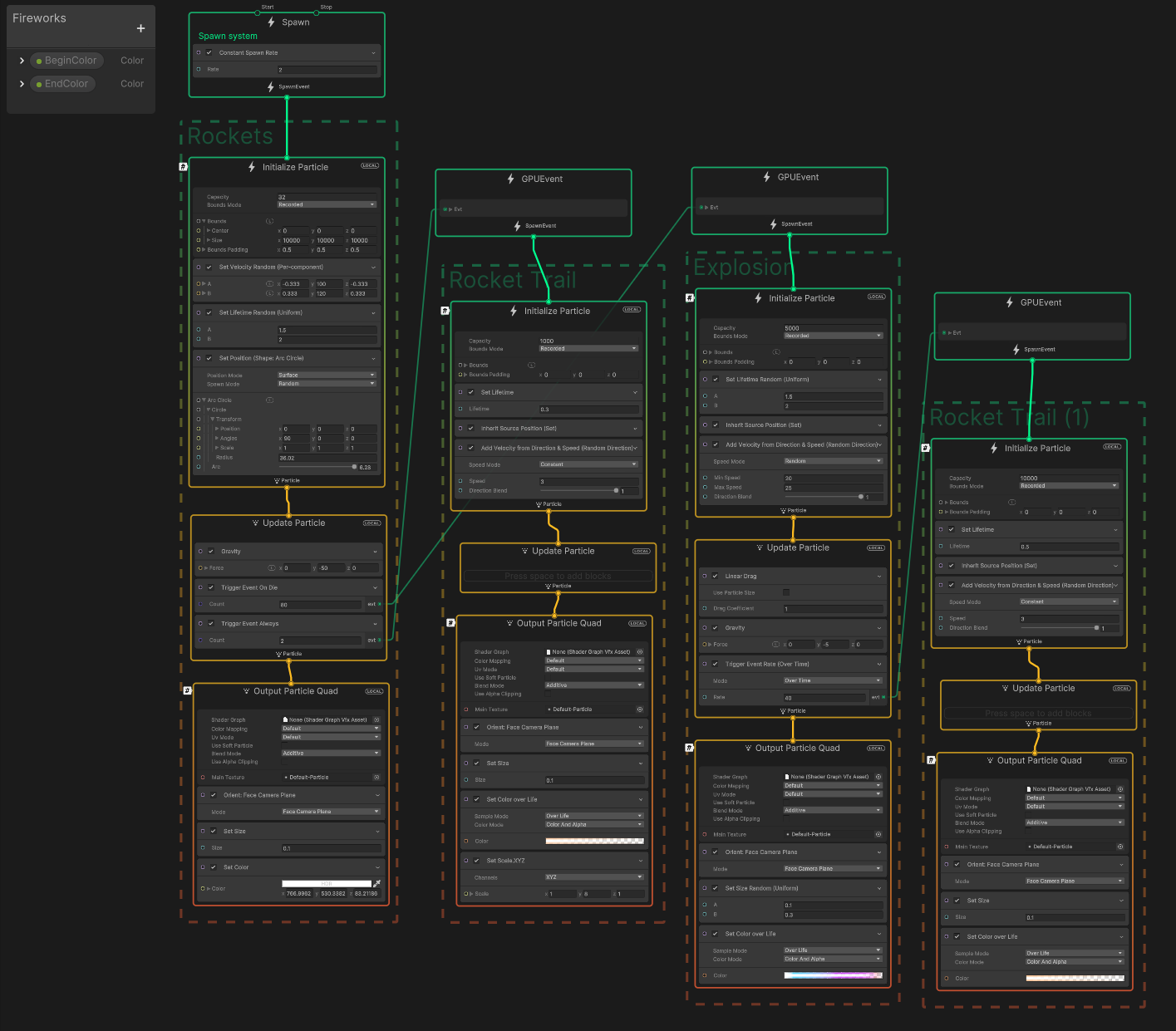
Particles system design in Unity Visual Effects Graph
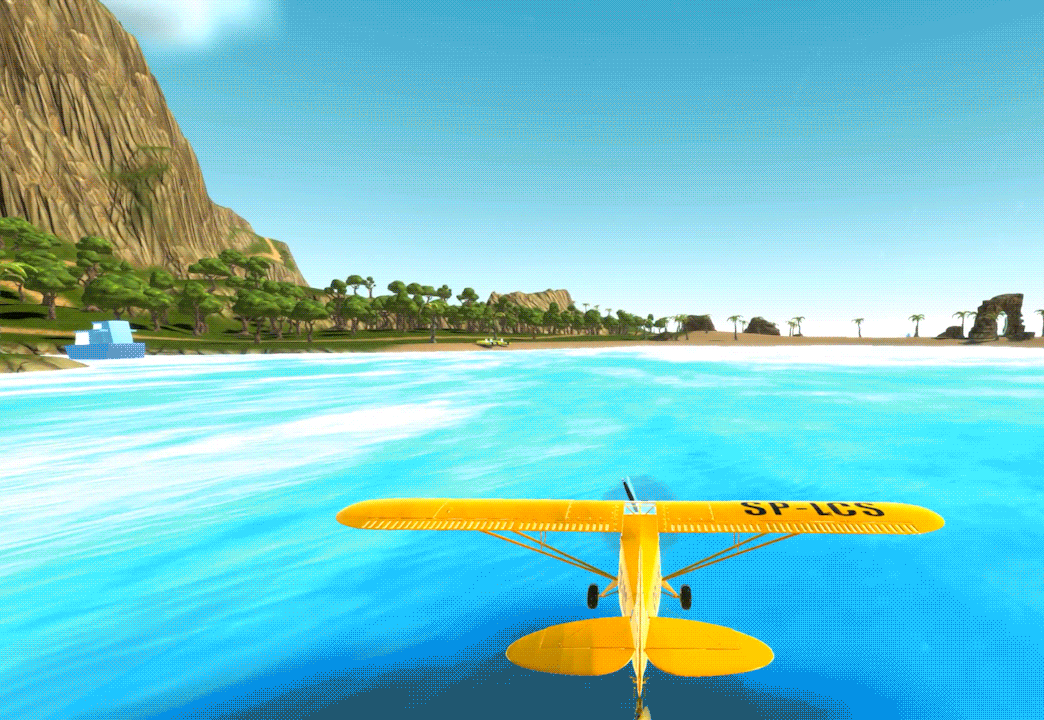
Stylized water splash effects with particles system